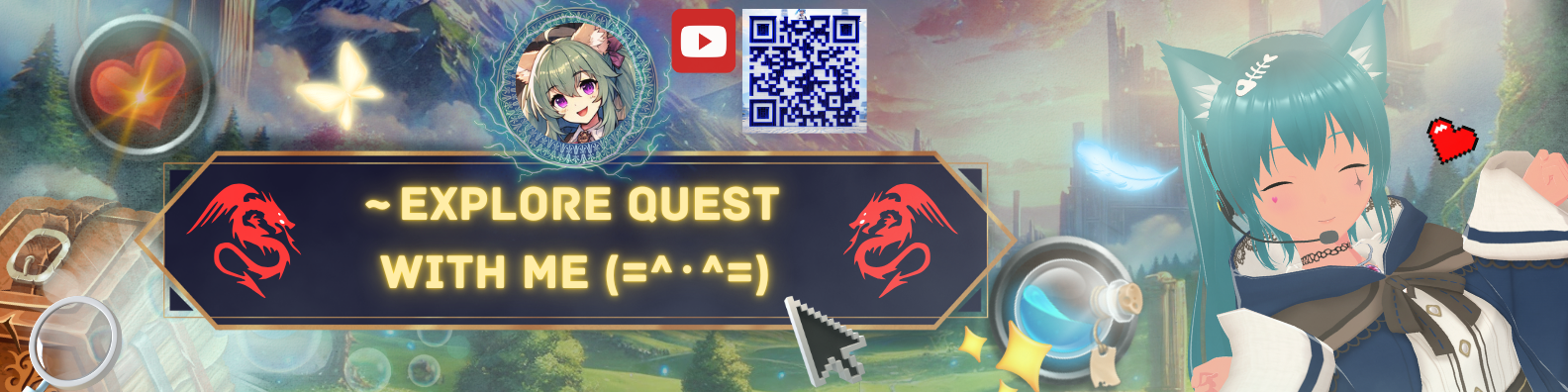
Master Ren’Py Menus & Unlock Interactive Choices!
A downloadable asset pack for Windows, macOS, and Linux
Master Ren’Py Menus & Unlock Choices – Full Source Code & Guide
~Meoww! Welcome, my dear code adventurers! ✨🐾(=^・^=)🩵💌 Mia here! In this Ren’Py tutorial, I’ll guide you step by step on how to create interactive choices using menus, variables, and conditions! Let’s unlock some coding magic together!
Here's what you'll learn:
📜 Define variables
🔐 Create choices with conditions
🛑 Prevent infinite item pickups
🚪 Unlock paths dynamically
How to Use the Source Code: 💾
A 🔹Download the full project or copy the source code at the bottom of this page.
B🔹If you downloaded the project, unzip the file and add it to your Ren’Py projects folder.
If you copy-paste the code, paste it into script.rpy in an existing or new Ren’Py project.
C 🔹Run your game and test your choices!
And now...~Let’s start our adventure, nyaaa!
1️⃣ Setting Up the Game Environment 🛠️
Defining Variables in init python 🔧🐾 (=^・^=) 🐍✨
Before we begin, we need to set up a variable to track whether the player has collected the key.
# Initializing Python init python: # Key not collected, so False. hasKey = False
📌 ~Why use a boolean variable here? ✅❌/ᐠ - ˕ •マ🔘💡
A boolean (True / False) makes it easy to check if the player has the key or not! If False, the player hasn’t picked it up yet; if True, they have. We’ll soon see how to update this value dynamically to change the course of the adventure!
Customization Tip: You can name this variable whatever you like, as long as you use the same name throughout your script to check and update its status!
2️⃣ Creating Interactive Choices with Conditions
📝 Using Menus to Let Players Choose! 🎮🐾(=^・^=)📜🔘
Now that we have our hasKey variable, let's create a menu where players can make decisions!
In Ren’Py, menus allow players to interact with the game by choosing different options. But what if we want some choices to appear only if a condition is met? That’s where our if statements come in!
# Start of the adventure with the start label label start: # Menu to choose the action menu: # ~If adventurers have the key, they can open the door. "Try to open the door." if hasKey: # If they open the door, jump to the door scene. jump door_scene # ~If they don't have the key yet, they can pick it up. "Pick up the key": # Jump to the key-picking action. jump pick_up_key
📌 Why use conditions in menus? 🔍✨/ᐠ - ˕ •マ📜
Conditions let you lock or unlock choices dynamically, making the game feel more interactive! In this case, we’ll hide the "Open the door" option unless the player has the key!
Customization Tip: You can use conditions for any kind of game logic, not just keys! For example, show a special dialogue only if the player has met a character before, or unlock a new area after completing a quest! Just swap hasKey with any variable you need!
➡️ Next, let’s make sure players can pick up the key...~Without collecting infinite keys, meow!
3️⃣ Preventing Infinite Pickups 🛑
🗝️ Using If Checks to Prevent Repeating Actions 🔄✨/ᐠ - ˕ •マ🛑🎮
Now that we let players pick up the key, we need to make sure they can’t collect it infinitely!
# Label for the action of picking up the key label pick_up_key: # If the meowww key hasn't been picked up yet... if hasKey == False: # Pick up the key, set the variable to True. $hasKey = True # Display a confirmation message. "You pick up the key!" # If the key has already been picked up... else: # Display that the key is already taken. "You already picked up the key." # Return to the main menu. jump start
📌 Why use an if check here? ✅🔄/ᐠ - ˕ •マ💡
Without a condition, the player could grab the key over and over, breaking the game logic! Using an if statement, we only allow them to pick it up once...~and if they already have it, we show a different message!
Customization Tip: You can use this same method for any limited item! Potions, coins, weapons, or even story triggers! Just replace hasKey with another variable like hasPotion or foundSecret, and control when players can interact!
➡️ Next, let's see how to use the key to unlock the door!
4️⃣ Unlocking Door & Returning to the Menu 🚪
🔑 Using Labels & Return to Control Game 🔄🐾(=^・^=)✨🩵
Now that we’ve picked up the key, it’s time to unlock the door!
# Label for the action of opening the door label door_scene: # ~Congratulations, the door is unlocked! "You unlock the door with your key!" # Return to the main menu, nyaaa! return
📌 Why use return here? 🔄🗨️/ᐠ - ˕ •マ✨
Once the player unlocks the door, we don’t want the game to continue endlessly in this scene. The return command ends the label and sends the player back to the menu, keeping the game structured!
Customization Tip: You can use return in any label or screen to bring the player back to a previous point! Need to close a popup? Finish a side quest? Use return to keep things clean and organized!
➡️ Now, let's wrap up everything in our final step!
Wrapping Up: Mastering Menus in Ren’Py! 📚
And that’s it, meoww! You’ve now learned how to create interactive menus, use conditions to unlock choices, prevent infinite pickups, and control scene flow with return! 🔄💡/ᐠ > ˕ <マ🎉💖
With just a few lines of code, you can guide the player’s choices dynamically, making your game more engaging and fun!
Quick Recap of This Little Quest:
- Define variables to track player progress.
- Use menus to let players make choices.
- Add if conditions to unlock actions only when needed.
- Use return to manage scene flow smoothly.
Customization Tip: Play around with variables! Try adding more conditions, new objects to collect, or even a secret door that only opens under special conditions! Who knows what exciting adventures you'll create?
A huge thank you for reading this tutorial and for your pawsome support, nyaaa! Feel free to share, remix, and build upon it to create your own Ren’Py adventures! If this tutorial helped you, please follow me for free on my social networks and YouTube channel! ~It really means a lot to me and helps me keep creating, ~thank you!
If you ever need anything, don’t hesitate to reach me out! I’d be super excited to help you on your coding journey! 🐾✨(^u^)✨💓
Happy coding & see you next time! Love youuu, and have a meoww blessed day!
~Mia 📖✨🐾(≧◡≦)💖🌟
Source Code:
# Initializing Python init python: # Key not collected, so False. hasKey = False # Start of the adventure with the start label label start: # Menu to choose the action menu: # ~If adventurers have the key, they can open the door. "Try to open the door." if hasKey: # If they open the door, jump to the door scene. jump door_scene # ~If they don't have the key yet, they can pick it up. "Pick up the key": # Jump to the key-picking action. jump pick_up_key # Label for the action of picking up the key label pick_up_key: # If the meowww key hasn't been picked up yet... if hasKey == False: # Pick up the key, set the variable to True. $hasKey = True # Display a confirmation message. "You pick up the key!" # If the key has already been picked up... else: # Display that the key is already taken. "You already picked up the key." # Return to the main menu. jump start # Label for the action of opening the door label door_scene: # ~Congratulations, the door is unlocked! "You unlock the door with your key!" # Return to the main menu, nyaaa! return
~Still here? Thank you sooo much for your support! ~Love youu!
Status | Released |
Category | Assets |
Author | Discover with Mia |
Genre | Visual Novel |
Tags | Fantasy, Ren'Py, sourcecode, Tutorial |
Leave a comment
Log in with itch.io to leave a comment.